Tags
- LV2
- DP
- ๊น์ด ์ฐ์ ํ์
- ๊ตฌํ
- CodingTest
- ์ํ
- ๊ทธ๋ํ ํ์
- ์ ๋ ฌ
- ๊ต์ฌ
- stack
- ์๋ฃ๊ตฌ์กฐ
- dfs
- Python
- Java
- Study
- BFS
- ์๋ฎฌ๋ ์ด์
- ๋๋น ์ฐ์ ํ์
- Dynamic Programming
- sort
- ์ ์๋ก
- BOJ
- greedy
- PGM
- ๊ทธ๋ํ ์ด๋ก
- ๋ฐฑํธ๋ํน
- Brute Force Algorithm
- SpringBoot
- ๋ฌธ์์ด
- queue
Archives
๊ธฐ๋ก๋ฐฉ
BOJ_3190 : ๋ฑ ๋ณธ๋ฌธ
3190๋ฒ: ๋ฑ
'Dummy' ๋ผ๋ ๋์ค๊ฒ์์ด ์๋ค. ์ด ๊ฒ์์๋ ๋ฑ์ด ๋์์ ๊ธฐ์ด๋ค๋๋๋ฐ, ์ฌ๊ณผ๋ฅผ ๋จน์ผ๋ฉด ๋ฑ ๊ธธ์ด๊ฐ ๋์ด๋๋ค. ๋ฑ์ด ์ด๋ฆฌ์ ๋ฆฌ ๊ธฐ์ด๋ค๋๋ค๊ฐ ๋ฒฝ ๋๋ ์๊ธฐ์์ ์ ๋ชธ๊ณผ ๋ถ๋ชํ๋ฉด ๊ฒ์์ด ๋๋๋ค. ๊ฒ์
www.acmicpc.net
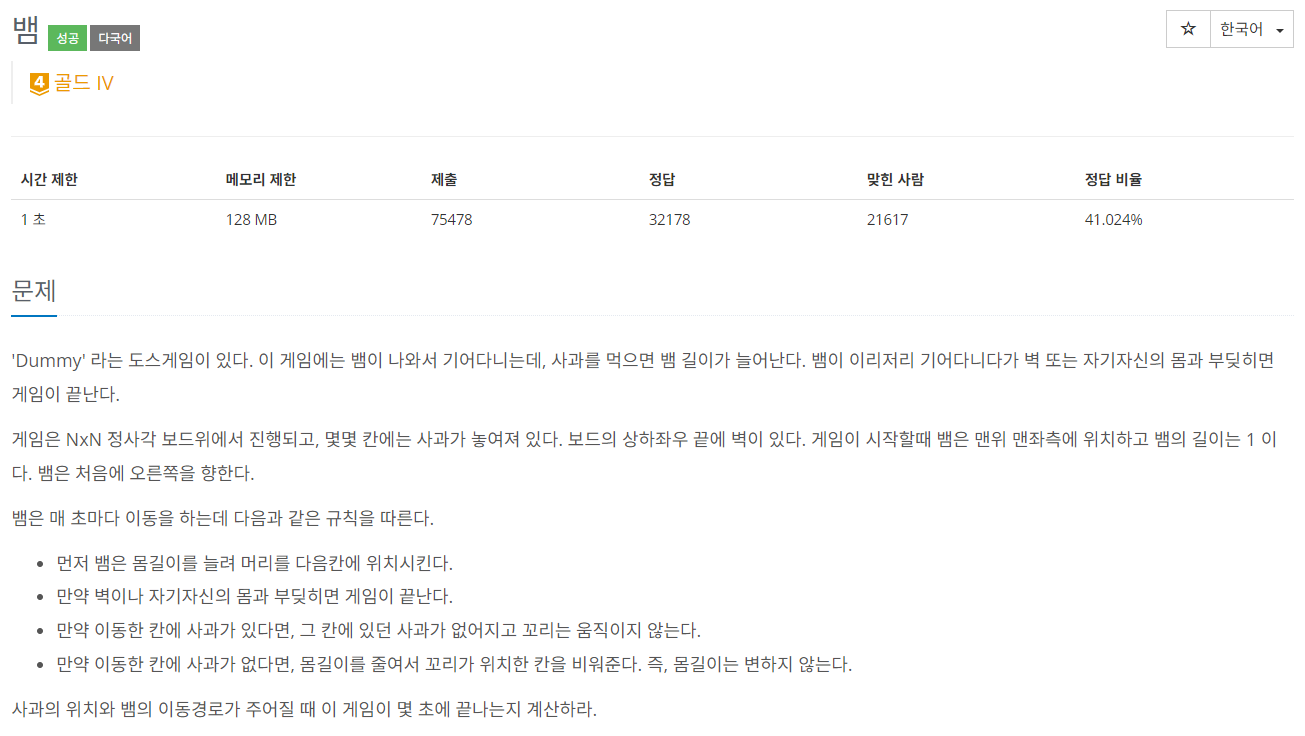
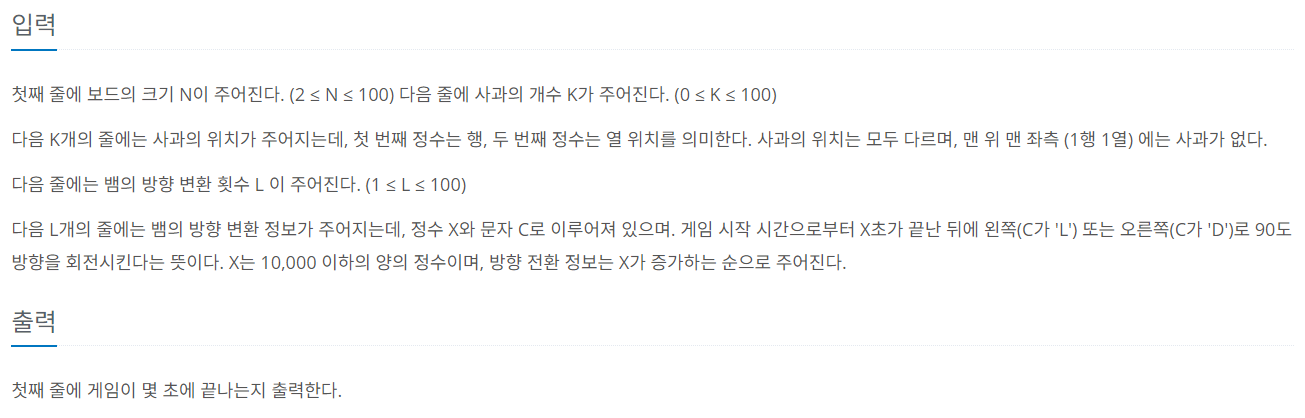
๐ธ ๋ฌธ์ ๋ถ์ ๐ธ
- NxN ๊ฒ์ํ์์ ๋ฑ์ด ์ด๋ํ๋ ๊ฒ์์ด ๋ช์ด๊ฐ ์งํ ๋๋์ง ๋ฐํํ๋ค.
- ๊ฒ์ ํ์๋ K๊ฐ์ ์ฌ๊ณผ๊ฐ ์๊ณ , ์ฌ๊ณผ๋ฅผ ๋จน์ผ๋ฉด ๋ชธ ๊ธธ์ด๊ฐ 1 ๋์ด๋๋ฉฐ ๊ธฐ์กด์ ๊ผฌ๋ฆฌ ์์น๊ฐ ๋ณ๊ฒฝ๋์ง ์๋๋ค.
- ๋ชธ ํน์ ๊ฒ์ํ ๋ฒฝ์ ๋ถ๋ชํ๋ฉด ๊ฒ์์ด ์ข ๋ฃ๋๋ค.
๐ธ ๋ฌธ์ ํ์ด ๐ธ
- ํน๋ณํ ์๊ณ ๋ฆฌ์ฆ์ด ์๋ ์๋ฎฌ๋ ์ด์ ๋ฌธ์ ์ด๋ค.
- ๋ฑ์ ๋ฐฉํฅ ์ ํ ์ ๋ณด์ ๋ฑ์ ๊ผฌ๋ฆฌ ์ขํ๋ฅผ ๊ธฐ์ตํ๊ธฐ ์ํด ํ ์๋ฃ๊ตฌ์กฐ๋ฅผ ์ฌ์ฉํ๋ค.
๐ธ ์ฝ๋ ๐ธ
import java.io.*;
import java.util.ArrayDeque;
import java.util.Queue;
import java.util.StringTokenizer;
public class Main {
public static void main(String[] args) throws IOException {
// Input
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
BufferedWriter bw = new BufferedWriter(new OutputStreamWriter(System.out));
StringTokenizer st = null;
byte N = Byte.parseByte(br.readLine());
byte[][] board = new byte[N + 1][N + 1]; // ๊ฒ์ํ
byte K = Byte.parseByte(br.readLine());
for (int i = 0; i < K; i++) { // ์ฌ๊ณผ ์์น ๊ธฐ๋ก : 1
st = new StringTokenizer(br.readLine());
board[Byte.parseByte(st.nextToken())][Byte.parseByte(st.nextToken())] = 1;
}
byte L = Byte.parseByte(br.readLine());
Queue<Turn> que = new ArrayDeque<>(); // ํ์ ์ ๋ณด ์ ์ฅ
for (int i = 0; i < L; i++) { // ํ์ ์ ๋ณด ๊ธฐ๋ก
st = new StringTokenizer(br.readLine());
que.add(new Turn(Short.parseShort(st.nextToken()), st.nextToken().charAt(0) == 'L' ? (byte) 3 : (byte) 1));
}
// Play
int answer = playDummy(board, que);
// Output
bw.write(Integer.toString(answer));
bw.flush();
}
private static int playDummy(byte[][] board, Queue<Turn> que) {
// ๋ฐฉํฅ ์ ๋ณด ์ด๊ธฐํ
int[] dx = {-1, 0, 1, 0};
int[] dy = {0, 1, 0, -1};
int direction = 1; // ๋ฑ์ ์ค๋ฅธ์ชฝ์ ๋ณด๊ณ ์์
// ์ขํ ์ ๋ณด ์ด๊ธฐํ
Position now = new Position(1, 1);
board[1][1] = 2;
// ๊ผฌ๋ฆฌ ์ ๋ณด
Queue<Position> tail = new ArrayDeque<>();
tail.add(new Position(1, 1));
Turn turn = que.poll();
int time = 0;
while (true) {
// ์๊ฐ ์ฆ๊ฐ
time++;
// ์ด๋
now.row += dx[direction];
now.col += dy[direction];
// ๋ฐฉํฅ ๋ณ๊ฒฝ
if (time == turn.X) {
direction = (direction + turn.C) % 4;
if (!que.isEmpty()) {
turn = que.poll();
}
}
// ๊ฒ์ํ ๋ฒ์ด๋๋ฉด ์ข
๋ฃ
if (now.row <= 0 || board.length <= now.row || now.col <= 0 || board[0].length <= now.col) {
return time;
}
if (board[now.row][now.col] == 0) { // ์ผ๋ฐ ์ด๋
board[now.row][now.col] = 2;
if (!tail.isEmpty()) { // ๊ผฌ๋ฆฌ ์์ฐ
Position tp = tail.poll();
board[tp.row][tp.col] = 0;
}
tail.add(new Position(now.row, now.col));
} else if (board[now.row][now.col] == 1) { // ์ฌ๊ณผ ๋จน์
board[now.row][now.col] = 2;
tail.add(new Position(now.row, now.col));
} else { // ๋ฑ ๋ชธ์ ๋ถ๋ชํ
return time;
}
}
}
private static class Position {
int row, col;
public Position(int row, int col) {
this.row = row;
this.col = col;
}
}
private static class Turn {
short X;
Byte C;
public Turn(short x, Byte c) {
X = x;
C = c;
}
}
}
๐ธ ์ฝ๋ ํด์ ๐ธ
- ์ฝ๋์ ํ๋ฆ์ ์ฃผ์์ผ๋ก ์ ์ ๋ฆฌ๋์ด ์์ผ๋, ๊ฐ๋จํ ํ๋ฆ๋ง ์ค๋ช
ํ๋ค.
- ๊ฒ์ํ์ ์ฌ๊ณผ ์์น ์ ๋ณด๋ฅผ ๊ธฐ๋กํ๋ค.
- ๋ฐฉํฅ ์ ํ ์ ๋ณด๋ฅผ ํ์ ๋ฃ๋๋ค.
- ๊ฒ์์ ์งํํ๋ฉฐ ๊ผฌ๋ฆฌ ์ขํ๋ฅผ ํ์ ๋ฃ๋๋ค.
- ๊ฒ์ํ์ ๋ฒ์ด๋๊ฑฐ๋ ๋ชธ์ ๋ถ๋ชํ๋ฉด ๊ฒ์์ ์ข ๋ฃํ๋ค.
- ์ด๋ ํ์ ๋ฐฉํฅ์ ๋ณ๊ฒฝํ๋ ์ ์ ์ ์ํ๋ค.
๐ธ end ๐ธ
- ๋จ์ ๊ตฌํ ๋ฌธ์ ์ฌ์ ํฌ๊ฒ ์ด๋ ค์ด ๋ถ๋ถ์ ์์๋ค.
- ๋ฌธ์ ์์ ์์ธํ ์ค๋ช ๋์ด ์ง์ง ์์ ๊ฒ ๊ฐ์๋ฐ, ๋ฑ์ ์ด๋ ์ดํ์ ๋ฐฉํฅ์ด ๋ณ๊ฒฝ๋๋ ๋ถ๋ถ์ด ์์ด์ ํ ์คํธ ์ผ์ด์ค๋ฅผ ๋ณด๊ณ ์กฐ๊ธ ๋๋ฒ๊น ํ ํ์๊ฐ ์์๋ค.
- ์
๋ ฅ ๊ฐ์ด 100 ์ดํ์ธ ๋ถ๋ถ์ด ๋ง์์ ๊ฐ์๊ธฐ ๋ฉ๋ชจ๋ฆฌ๋ฅผ ์๊ปด์ ๊ตฌํํด ๋ณด๊ณ ์ถ์๋ค.
- -128~127 ๊น์ง ์ฌ์ฉ ๊ฐ๋ฅํ Byte์ X๋ง ๊ฐ์ด ์ปค์ Short๋ฅผ ์ฌ์ฉํด์ ๊ตฌํํด๋ณด์๋ค.
- ํ์ง๋ง NumberFormatException์ด ๋ ์ ๊ทธ๋ฅ ์ ๋ถ int๋ก ๋ฐ๊ฟ๋ณด๋ ํต๊ณผ ๋์๋ค.
- ๋ญ๊ฐ ๋ฌธ์ ์ธ๊ฐ ๋ค์ ์ดํด๋ณด๋ X๋ง Shortํ์ธ๋ฐ Byte๋ก ์ ๋ ฅ์ ๋ณํํ๊ฒ ๋ฌธ์ ์๋ค.
- ์ญ์ ์ฝ๋ฉ ํ ์คํธ์์๋ ์ด๋ ๊ฒ ๋ฉ๋ชจ๋ฆฌ๋ฅผ ์๋ผ์ง ์์๋ ๋๋ int ํน์ long์ ์ฐ๋๊ฒ ๋ง๋ ๊ฒ ๊ฐ๋ค.

728x90
'CodingTest > Java' ์นดํ ๊ณ ๋ฆฌ์ ๋ค๋ฅธ ๊ธ
BOJ_2580 : ์ค๋์ฟ (0) | 2024.04.19 |
---|---|
BOJ_1707 : ์ด๋ถ ๊ทธ๋ํ (0) | 2024.04.13 |
BOJ_2143 : ๋ ๋ฐฐ์ด์ ํฉ (0) | 2024.04.10 |
BOJ_1644 : ์์์ ์ฐ์ํฉ (0) | 2024.04.09 |
BOJ_12015 : ๊ฐ์ฅ ๊ธด ์ฆ๊ฐํ๋ ๋ถ๋ถ ์์ด 2 (0) | 2024.04.09 |